User Interface Architecture
MIDP 2.0 provides UI classes in two packages, javax.microedition.lcdui
and javax.microedition.lcdui.
A D V E R T I S E M E N T
game, where lcdui stands
for liquid crystal display user interface (LCD UI). As expected, the game
package contains classes for development of a wireless game UI. I will discuss
this package in the next part of this series.
The UI classes of of MIDP 2.0's javax.microedition.lcdui package
can be divided into two logical groups: the high- and low-level
groups. The classes of the high-level group are perfect for development of
MIDlets that target the maximum number of devices, because these classes do not
provide exact control over their display. The high-level classes are heavily
abstracted to provide minimal control over their look and feel, which is left
for device on which they are deployed to manage, according to its capabilities.
These classes are shown in Figure 1.
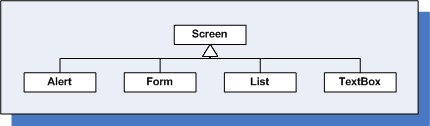
Figure 1. High-level MIDP 2.0 UI classes
The classes of the low-level group are perfect for MIDlets where precise
control over the location and display of the UI elements is important and
required. Of course, with more control comes less portability. If your MIDlet is
developed using these classes, it may not be deployable on certain devices,
because they require precise control over the way they look and feel. There are
only two classes in this group, and they are shown in Figure 2.
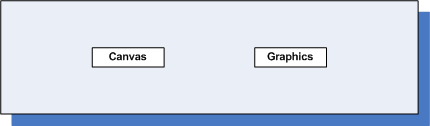
Figure 2. Low-level MIDP 2.0 UI classes
There is another class in the low-level group called GameCanvas,
which is not shown here, as it will be discussed in the next part of this
series.
For you to be able to show a UI element on a device screen, whether high- or
low-level, it must implement the Displayable interface. A
displayable class may have a title, a ticker, and certain commands associated
with it, among other things. This implies that both the Screen and
Canvas classes and their subclasses implement this interface, as
can be seen in Figure 3. The Graphics class does not implement this
interface, because it deals with low-level 2D graphics that directly manipulate
the device's screen.
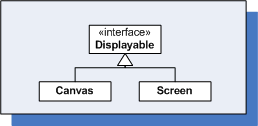
Figure 3. Canvas and Screen implement the
Displayable interface
A Displayable class is a UI element that can be shown on the
device's screen while the Display class abstracts the display
functions of an actual device's screen and makes them available to you. It
provides methods to gain information about the screen and to show or change the
current UI element that you want displayed. Thus, a MIDlet shows a
Displayable UI element on a Display using the
setCurrent(Displayable element) method of the Display class.
As the method name suggests, the Display can have only one
Displayable element at one time, which becomes the current element on
display. The current element that is being displayed can be accessed using the
method getCurrent(), which returns an instance of a
Displayable element. The static method getDisplay(MIDlet midlet)
returns the current display instance associated with your MIDlet method.
A little bit of actual code here would go a long way in helping understand
the MIDlet UI concepts that we have just discussed. Rather than write new code,
let's try and retrofit our understanding on the Date-Time MIDlet example from
part one, which is reproduced in Listing 1.
package com.j2me.part1;
import java.util.Date;
import javax.microedition.lcdui.Alert;
import javax.microedition.lcdui.Display;
import javax.microedition.midlet.MIDlet;
public class DateTimeApp extends MIDlet {
Alert timeAlert;
public DateTimeApp() {
timeAlert = new Alert("Alert!");
timeAlert.setString(new Date().toString());
}
public void startApp() {
Display.getDisplay(this).setCurrent(timeAlert);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
Listing 1. DateTimeApp MIDlet
A Displayable UI element, an Alert, is created in
the constructor. When the device's Application Management Software (AMS) calls
the startApp() method, the current display available for this
MIDlet is extracted using the Display.getDisplay() method. The
Alert is then made the current item on display, by setting it as a
parameter to the setCurrent() method.
As seen from Figure 1, there are four high-level UI elements that can be
displayed on a MIDlet's screen. Let's discuss each of these elements in detail.
|