Handling User Commands
None of the UI elements so far have allowed any interaction from the user! A
MIDlet interacts with a user through commands.
A D V E R T I S E M E N T
A command is the equivalent of a
button or a menu item in a normal application, and can only be associated with a
displayable UI element. Like a ticker, the Displayable class allows
the user to attach a command to it by using the method addCommand(Command
command). Unlike a ticker, a displayable UI element can have multiple
commands associated with it.
The Command class holds the information about a command. This
information is encapsulated in four properties. These properties are: a short
label, an optional long label, a command type, and a priority. You create a
command by providing these values in its constructor:
Command exitCommand = new Command("EXIT", Command.EXIT, 1);
Note that commands are immutable once created.
By specifying the type of a command, you can let the device running the
MIDlet map any predefined keys on the device to the command itself. For example,
a command with the type OK will be mapped to the device's OK
key. The rest of the types are: BACK, CANCEL,
EXIT, HELP, ITEM, SCREEN, and
STOP. The SCREEN type relates to an
application-defined command for the current screen. Both SCREEN and
ITEM will probably never have any device-mapped keys.
By specifying a priority, you tell the AMS running the MIDlet where and how
to show the command. A lower value for the priority is of higher importance, and
therefore indicates a command that the user should be able to invoke directly.
For example, you would probably always have an exit command visible to the user
and give it a priority of 1. Since the screen space is limited, the device then
bundles less-important commands into a menu. The actual implementation varies
from device to device, but the most likely scenario involves one priority-1
command displayed along with an option to see the other commands via a menu.
Figure 7 shows this likely scenario.
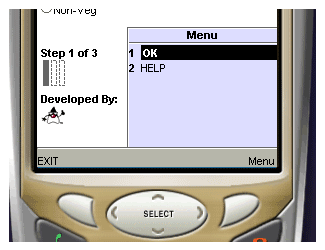
Figure 7. The way commands are displayed. The menu pops up when the user
presses the key corresponding to the menu command.
The responsibility for acting on commands is performed by a class
implementing the CommandListener interface, which has a single
method: commandAction(Command com, Displayable dis). However,
before command information can travel to a listener, The listener is registered
with the method setCommandListener(CommandListener listener) from
the Displayable class.
Putting this all together, Listing 6 shows how to add some commands to the
form discussed in Listing 4.
package com.j2me.part2;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.Gauge;
import javax.microedition.lcdui.Spacer;
import javax.microedition.lcdui.ImageItem;
import javax.microedition.lcdui.TextField;
import javax.microedition.lcdui.DateField;
import javax.microedition.lcdui.StringItem;
import javax.microedition.lcdui.ChoiceGroup;
import javax.microedition.lcdui.Image;
import javax.microedition.lcdui.Choice;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Command;
import javax.microedition.midlet.MIDlet;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.CommandListener;
public class FormExample
extends MIDlet
implements CommandListener {
private Form form;
private Gauge gauge;
private Spacer spacer;
private ImageItem imageItem;
private TextField txtField;
private DateField dateField;
private StringItem stringItem;
private ChoiceGroup choiceGroup;
public FormExample() {
form = new Form("Your Details");
// a StringItem is not editable
stringItem = new StringItem("Your Id: ", "WXP-890");
form.append(stringItem);
// you can accept Date, Time or DateTime formats
dateField =
new DateField("Your DOB: ", DateField.DATE);
form.append(dateField);
// similar to using a TextBox
txtField = new TextField(
"Your Name: ", "", 50, TextField.ANY);
form.append(txtField);
// similar to using a List
choiceGroup = new ChoiceGroup(
"Your meals: ",
Choice.EXCLUSIVE,
new String[] {"Veg", "Non-Veg"},
null);
form.append(choiceGroup);
// put some space between the items
spacer = new Spacer(20, 20);
form.append(spacer);
// a gauge is used to show progress
gauge = new Gauge("Step 1 of 3", false, 3, 1);
form.append(gauge);
// an image may not be found,
// therefore the Exception must be handled
// or ignored
try {
imageItem = new ImageItem(
"Developed By: ",
Image.createImage("/duke.gif"),
ImageItem.LAYOUT_DEFAULT,
"Duke");
form.append(imageItem);
} catch(Exception e) {}
// create some commands and add them
// to this form
form.addCommand(
new Command("EXIT", Command.EXIT, 2));
form.addCommand(
new Command("HELP", Command.HELP, 2));
form.addCommand(
new Command("OK", Command.OK, 1));
// set itself as the command listener
form.setCommandListener(this);
}
// handle commands
public void commandAction(
Command com, Displayable dis) {
String label = com.getLabel();
if("EXIT".equals(label))
notifyDestroyed();
else if("HELP"Equals(label))
displayHelp();
else if("OK"Equals(label))
processForm();
}
public void displayHelp() {
// show help
}
public void processForm() {
// process Form
}
public void startApp() {
Display display = Display.getDisplay(this);
display.setCurrent(form);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
Listing 6. Adding commands to a form
The differences from Listing 4 are highlighted in bold. The command listener
in this case is the form class itself, and therefore, it implements the
commandAction() method. Note that this method also accepts a displayable
parameter, which is very useful. Because commands are immutable, they can be
attached to multiple displayable objects, and this parameter can help
distinguish which displayable object invoked the command.
|