Building a J2ME Game: Creating Backgrounds Using the
TiledLayer Class
A D V E R T I S E M E N T
In this section, you will add some color to the game by providing a
background using the TiledLayer class. The game is divided into
three sections: the top section can be thought of as the sky, the middle section
in which the couple jump is the earth, and the bottom section is the sea. These
three sections can be filled easily using three colored images of the size 32 by
32 pixels each, one for each section. However, each section is bigger than 32 by
32 pixels, and the TiledLayer class is used to define large areas
like these with small images.
To start, divide the game screen into squares of 32 by 32 each and number
each row and column, starting with an index of 0. This is shown in Figure 4 and
results in a 5-by-5-cell background.
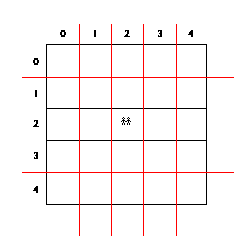
Figure 4. Divide the game screen into individual cells
Thus, cells (0, 0) to (1, 4) are to painted with a sky image; cells (2, 0) to
(2, 4) are to be painted with an earth image, and cells (3, 0) to (4, 4) are to
be painted with a sea image. You will do this with the image shown in Figure 5.

Figure 5. Background image
The first 32 by 32 cell represents the earth image, the second represents the
sea, and the last represents the sky. When you use the TiledLayer
class, these images are numbered starting from index 1 (not 0; therefore, earth
is 1, sea is 2, and sky is 3). The TiledLayer class will take this
one image and divide it into three separate images used for rendering the game
background. In our case, we want the TiledLayer class to render a
5-by-5-cell background using cells of 32 by 32 pixels each. This is achieved by
the following code:
// load the image
backgroundImg = Image.createImage("/tiledLayer1.gif");
// create the tiledlayer background
background = new TiledLayer(5, 5, backgroundImg, 32, 32);
As you can see, the first two parameters to the TiledLayer
constructor represent the total background size, the next parameter represents
the image, and the last two parameters represents the size of each cell. This
size will be used by the TiledLayer class to carve the image into
its individual background cells.
All that is now left is to set each cell with its respective image. The full
code to create the background is listed below in a method called
createBackground(). You will need to add a call to this method from the
start() method of the MyGameCanvas class. Once this is
done, add a call to paint this background using background.paint(g)
at the end of the buildGameScreen() method, which will render it to
screen.
// creates the background using TiledLayer
private void createBackground() throws IOException {
// load the image
backgroundImg = Image.createImage("/tiledlayer1.gif");
// create the tiledlayer background
background = new TiledLayer(5, 5, backgroundImg, 32, 32);
// array that specifies what image goes where
int[] cells = {
3, 3, 3, 3, 3, // sky
3, 3, 3, 3, 3, // sky
1, 1, 1, 1, 1, // earth
2, 2, 2, 2, 2, // sea
2, 2, 2, 2, 2 // sea
};
// set the background with the images
for (int i = 0; i < cells.length; i++) {
int column = i % 5;
int row = (i - column)/5;
background.setCell(column, row, cells[i]);
}
// set the location of the background
background.setPosition(GAME_ORIGIN_X, GAME_ORIGIN_Y);
}
The final result will look like Figure 6.
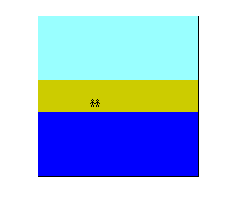
Figure 6. Game with a background added
|