Images, Tickers, and Gauges
Using images, tickers, and gauges as UI elements in MIDlets is quite
straightforward.
A D V E R T I S E M E N T
A gauge, as you saw in the last section, is an item that can
only be displayed on a form to indicate progress or to control a MIDlet feature
(like volume). A ticker, on the other hand, can be attached to any UI element
that extends the Displayable abstract class, and results in a
running piece of text that is displayed across the screen whenever the element
that is attached to it is shown on the screen. Finally, an image, can be used
with various UI elements, including a form, as we saw in the last section.
Since the ticker can be used with all displayable elements, it provides a
handy way to display information about the current element on the screen. The
Displayable class provides the method setTicker(Ticker
ticker), and the ticker can itself be created using its constructor
Ticker(String msg), with the message that you want the ticker to display.
By using setString(String MSG), you can change this message, and
this change is effected immediately. For example, the form used in the previous
section can have its own ticker displayed by setting form.setTicker(new
Ticker("Welcome to Vandalay Industries!!!")). This will result in a
ticker across the top of the screen (in the Toolkit's emulator), while the user
is filling out the form. This is shown in Figure 6.
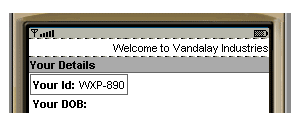
Figure 6. Setting a Ticker on a Displayable
In the previous section, we saw an example of a gauge in a non-interactive
mode. It was there to represent the progress of a form being filled out by the
MIDlet user. A non-interactive gauge can be used to represent the progress of a
certain task; for example, when the device may be trying to make a network
connection or reading a datastore, or when the user is filling out a form. In
the previous section, we created a gauge by specifying four values. The label
("Step 1 of 3"), the interactive mode (false), the maximum value (3) and the
initial value (1). However, when you don't know how long a particular activity
is going to take, you can use the value of INDEFINITE for the
maximum value.
A non-interactive gauge that has an INDEFINITE maximum value
acquires special meaning. (You cannot create an interactive gauge with an
INDEFINITE maximum value.) This type of gauge can be in one of four
states, and this is reflected by the initial value (which is also the current
value of the gauge). These states are CONTINUOUS_IDLE,
INCREMENTAL_IDLE, CONTINUOUS_RUNNING, and
INCREMENTAL_UPDATING. Each state represents the best effort of the device
to let the user know the current activity of the MIDlet, and you can use them to
represent these states yourself. Listing 5 shows an example of using these
non-interactive gauges, along with an example of an interactive gauge. Remember
that a Gauge is a UI Item, and therefore, can only be
displayed as part of a Form.
Package com.j2me.part2;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.Gauge;
import javax.microedition.lcdui.Display;
import javax.microedition.midlet.MIDlet;
public class GaugeExample extends MIDlet {
private Form form;
private Gauge niIndefinate_CI;
private Gauge niIndefinate_II;
private Gauge niIndefinate_CR;
private Gauge niIndefinate_IU;
private Gauge interactive;
public GaugeExample() {
form = new Form("Gauge Examples");
niIndefinate_CI =
new Gauge(
"NI - Cont Idle",
false,
Gauge.INDEFINITE,
Gauge.CONTINUOUS_IDLE);
form.append(niIndefinate_CI);
niIndefinate_II =
new Gauge(
"NI - Inc Idle",
false,
Gauge.INDEFINITE,
Gauge.INCREMENTAL_IDLE);
form.append(niIndefinate_II);
niIndefinate_CR =
new Gauge(
"NI - Cont Run",
false,
Gauge.INDEFINITE,
Gauge.CONTINUOUS_RUNNING);
form.append(niIndefinate_CR);
niIndefinate_IU =
new Gauge(
"NI - Inc Upd",
false,
Gauge.INDEFINITE,
Gauge.INCREMENTAL_UPDATING);
form.append(niIndefinate_IU);
interactive =
new Gauge(
"Interactive ",
true,
10,
0);
form.append(interactive);
}
public void startApp() {
Display display = Display.getDisplay(this);
display.setCurrent(form);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
Listing 5. Using Gauges
Each mobile device will use its own set of images to represent these gauges.
This will, in all probability, be different from the gauges that you will see if
you run this listing in the Emulator supplied with the Toolkit.
You can also associate an image with an Alert and a Choice-based
UI element. When an image is created, either by reading from a physical location
or by making an image in-memory, it exists only in the off-screen memory. You
should, therefore, be careful while using images, and restrict the size of
images to the minimum possible to avoid filling the device's available memory.
The Image class provides several static methods to create or
acquire images for use in MIDlets. An image that is created in-memory, by using
the createImage(int width, int height) method, is mutable, which
means that you can edit it. An image created this way initially has all of its
pixels set to white, and you can acquire a graphics object on this image by
using the method getGraphics() to modify the way it is rendered on
screen. More about the Graphics object follows in the low-level API
section.
To acquire an immutable image, you can use one of two methods:
createImage(String imageName) or createImage(InputStream stream).
The first method is used for looking up images from an associated packaged .jar
file, while the second method is good for reading an image over a network. To
create an immutable image from in-memory data, you can either use
createImage(byte[] imageData, int imageOffset, int imageLength) or
createImage(Image source). The first method allows you to form an image
out of a byte array representation, while the second allows the creation of an
image from an existing image.
Note that the MIDlet specification mandates support for
the Portable Network Graphics format for
images. Thus, all devices that support MIDlets will display a *.png image. These
devices may support other formats, especially GIF and JPEG formats, but that is
not a guarantee.
You have already seen an example of acquiring an image in the section on
forms. In Listing 4, an image was wrapped up in an ImageItem class
so that it could be displayed in a form. The image was kept in the res
folder of the MIDlet for the Toolkit and the Emulator to find. The
createImage(String imageName) method uses the
Class.getResourceAsStream(String imageName) method to actually locate
this image. The Toolkit takes care of packaging this image in the right folder
when you create a ,jar file. In this case, this will be the top-level .jar
folder. Make sure that whenever you reference images in your MIDlets that the
images are kept in the right location. For example, if you want to keep all of
your images for a MIDlet in an image folder in the final packaged .jar
file, and not the top-level .jar folder, you will need to keep these images
under an image folder under the res folder itself. To reference
any of these images, you will need to ensure that you reference them via this
images folder. For example: createImage("/images/duke.gif");
will reference the image duke.gif under the images folder.
|