Working with the Low-Level API
The low-level API for MIDlets is composed of the Canvas and
Graphics classes (we will discuss the GameCanvas class
in the next article).
A D V E R T I S E M E N T
The Canvas class is abstract; you must create
your own canvases to write/draw on by extending this class and providing an
implementation for the paint(Graphics g) method, in which the
actual drawing on a device is done. The Canvas and Graphics
classes work together to provide low-level control over a device.
Let's start with a simple canvas. Listing 7 shows an example canvas that
draws a black square in the middle of the device screen.
package com.j2me.part2;
import javax.microedition.lcdui.Canvas;
import javax.microedition.midlet.MIDlet;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Graphics;
public class CanvasExample
extends MIDlet {
Canvas myCanvas;
public CanvasExample() {
myCanvas = new MyCanvas();
}
public void startApp() {
Display display = Display.getDisplay(this);
// remember, Canvas is a Displayable so it can
// be set on the display like Screen elements
display.setCurrent(myCanvas);
// force repaint of the canvas
myCanvas.repaint();
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
class MyCanvas extends Canvas {
public void paint(Graphics g) {
// create a 20x20 black square in the center
g.setColor(0x000000); // make sure it is black
g.fillRect(
getWidth()/2 - 10,
getHeight()/2 - 10,
20, 20);
}
}
Listing 7. Creating and displaying a Canvas
The class MyCanvas extends Canvas and overrides the
paint() method. Although this method is called as soon as the
canvas is made the current displayable element (by setCurrent(myCanvas)),
it is a good idea to call the repaint() method on this canvas soon
afterwards. The paint() method accepts a Graphics
object, which provides methods for drawing 2D objects on the device screen. For
example, in Listing 7, a black square is created in the middle of the screen
using this Graphics object. Notice that before drawing the square,
using the fillRect() method, the current color of the
Graphics object is set to black by using the method g.setColor().
This is not necessary, as the default color is black, but this
illustrates how to change it if you wanted to do so.
If you run this listing, the output on the emulator will be as shown in
Figure 8.
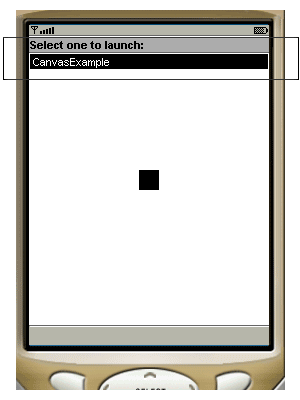
Figure 8. Drawing a single square in the middle of a Canvas
Notice the highlighted portion at the top in Figure 8. Even though the MIDlet
is running, the AMS still displays the previous screen. This is because in the
paint() method, the previous screen was not cleared away, and the
square was drawn on the existing surface. To clear the screen, you can add the
following code in the paint() method, before the square is drawn.
g.setColor(0xffffff);
// sets the drawing color to white
g.fillRect(0, 0, getWidth(), getHeight());
// creates a fill rect which is the size of the screen
Note that the getWidth() and getHeight() methods
return the size of the display screen as the initial canvas, which is the whole
display screen. Although the size of this canvas cannot be changed, you can
change the size and location of the clip area in which the actual rendering
operations are done. A clip area, in Graphics, is the area on which
the drawing operations are conducted. The Graphics class provides
the method setClip(int x, int y, int width, int height) to change
this clip area, which in an initial canvas is the whole screen, with the top
left corner as the origin (0, 0). Thus, if you use the method
getClipWidth() (or getClipHeight()) on the Graphics
object passed to the paint method in Listing 7, it returns a value equal to the
value returned by the getWidth() (or getHeight())
method of the Canvas.
The Graphics object can be used to render not only squares and
rectangles, but arcs, lines, characters, images, and text, as well. For example,
to draw the text "Hello World" on top of the square in Listing 7, you can add
the following code before or after the square is drawn:
g.drawString("Hello World", getWidth()/2, getHeight()/2 - 10,
Graphics.HCENTER | Graphics.BASELINE);
This will result in the screen shown in Figure 9.
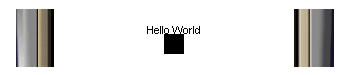
Figure 9. Drawing text using the Graphics object
Text, characters, and images are positioned using the concept of anchor
points. The full syntax of the drawString() method is
drawstring(String text, int x, int y, int anchor). The anchor positioning
around the x, y coordinates is specified by bitwise
ORing of two constants. One constant specifies the horizontal space
(LEFT, HCENTER, RIGHT) and the other
specifies the vertical space (TOP, BASELINE,
BOTTOM). Thus, to draw the "Hello World" text on top of the square, the
anchor's horizontal space needs to be centered around the middle of the canvas (getWidth()/2)
and hence, I have used the Graphics.HCENTER constant. Similarly,
the vertical space is specified by using the BASELINE constant
around the top of the square (getHeight()/2 - 10). You can also use
the special value of 0 for the anchor, which is equivalent to TOP | LEFT.
Images are similarly drawn and positioned on the screen. You can create
off-screen images by using the static createImage(int width, int height)
method of the Image class. You can get a Graphics
object associated with this image by using the getGraphics()
method. This method can only be called on images that are mutable. An image
loaded from the file system, or over the network, is considered an immutable
image, and any attempt to get a Graphics object on such an image
will result in an IllegalStateException at runtime.
Using anchor points with images is similar to using them with text and
characters. Images allow an additional constant for the vertical space,
specified by Graphics.VCENTER. Also, since there is no concept of a
baseline for an image, using the BASELINE constant will throw an
exception if used with an image.
Listing 8 shows the code snippet from the MyCanvas class
paint() method that creates an off-screen image, modifies it by adding an
image loaded from the file system, and draws a red line across it. Note that you
will need the image duke.gif in the res folder of the
CanvasExample MIDlet.
// draw a modified image
try {
// create an off screen image
Image offImg = Image.createImage(25, 19);
// get its graphics object and set its
// drawing color to red
Graphics offGrap = offImg.getGraphics();
offGrap.setColor(0xff0000);
// load an image from file system
Image dukeImg =
Image.createImage("/duke.gif");
// draw the loaded image on the off screen
// image
offGrap.drawImage(dukeImg, 0, 0, 0);
// and modify it by drawing a line across it
offGrap.drawLine(0, 0, 25, 19);
// finally, draw this modified off screen
// image on the main graphics screen
// so that it is just under the square
g.drawImage(
offImg, getWidth()/2,
getHeight()/2 + 10,
Graphics.HCENTER | Graphics.TOP);
} catch(Exception e) { e.printStackTrace(); }
Listing 8. Creating, modifying, and displaying an off-screen image on a
Canvas
The resultant screen, when combined with the "Hello World" text drawn
earlier, will look like Figure 10.
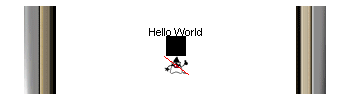
Figure 10. Text, a square, and a modified image drawn on a Canvas
The Canvas class provides methods to interact with the user,
including predefined game actions, key events, and, if a pointing device is
present, pointer events. You can even attach high-level commands to a canvas,
similar to attaching commands on a high-level UI element.
Each Canvas class automatically receives key events through the
invocation of the keyPressed(int keyCode), keyReleased(int
keyCode), and keyRepeated(int keyCode). The default
implementations of these methods are empty, but not abstract, which allows you
to only override the methods that you are interested in. Similar to the key
events, if a pointing device is present, pointer events are sent to the
pointerDragged(int x, int y), pointerPressed(int x, int y),
and pointerReleased(int x, int y) methods.
The Canvas class defines constants for key codes that are
guaranteed to be present in all wireless devices. These key codes define all of
the numbers (for example, KEY_NUM0, KEY_NUM1,
KEY_NUM2, and so on) and the star (*) and pound (#)
keys (KEY_STAR and KEY_POUND). This class makes it
even easier to capture gaming events by defining some basic gaming constants.
There are nine constants that are relevant to most games: UP,
DOWN, LEFT, RIGHT, FIRE,
GAME_A, GAME_B, GAME_C, and GAME_D.
But how does a key event translate to a gaming event?
By the use of the getGameAction() method. Some devices provide a
navigation control for moving around the screen, while some devices use the
number keys 2, 4, 6, and 8.
To find out which game action key was pressed, the Canvas class
encapsulates this information and provides it in the form of the game actions.
All you, as a developer, need to do is to grab the key code pressed by the user
in the right method, and use the getGameAction(int keyCode) method
to determine if the key pressed corresponds to a game action. As you can guess,
several key codes can correspond to one game action, but a single key code may
map to, at most, a single game action.
Listing 9 extends the original code from Listing 7 to add key code handling.
In this listing, the square in the middle of the screen is moved around with the
help of the navigation buttons.
Package com.j2me.part2;
import javax.microedition.lcdui.Canvas;
import javax.microedition.midlet.MIDlet;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Graphics;
public class CanvasExample
extends MIDlet {
Canvas myCanvas;
public CanvasExample() {
myCanvas = new MyCanvas();
}
public void startApp() {
Display display = Display.getDisplay(this);
// remember, Canvas is a Displayable so it can
// be set on the display like Screen elements
display.setCurrent(myCanvas);
// force repaint of the canvas
myCanvas.repaint();
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
class MyCanvas extends Canvas {
public void paint(Graphics g) {
// create a 20x20 black square in the center
// clear the screen first
g.setColor(0xffffff);
g.fillRect(0, 0, getWidth(), getHeight());
g.setColor(0x000000); // make sure it is black
// draw the square, changed to rely on instance variables
g.fillRect(x, y, 20, 20);
}
public void keyPressed(int keyCode) {
// what game action does this key map to?
int gameAction = getGameAction(keyCode);
if(gameAction == RIGHT) {
x += dx;
} else if(gameAction == LEFT) {
x -= dx;
} else if(gameAction == UP) {
y -= dy;
} else if(gameAction == DOWN) {
y += dy;
}
// make sure to repaint
repaint();
}
// starting coordinates
private int x = getWidth()/2 - 10;
private int y = getHeight()/2 - 10;
// distance to move
private int dx = 2;
private int dy = 2;
}
Listing 9. Handling key events to move the square
Notice that in this listing, the code to paint the square has been modified
to rely upon instance variables. The keyPressed() method has been
overridden and therefore, whenever the user presses a key, this method is
invoked. The code checks if the key pressed was a game key, and based on which
game key was pressed, changes the coordinates of the square accordingly.
Finally, the call to repaint() in turn calls the paint()
method, which moves the square on the screen as per the new coordinates.
In this article, you created the UI elements and were introduced to much of
the user interface APIs for MIDlets. In the next installment, you will learn to
use the Gaming API of MIDP 2.0 present in the package
javax.microedition.lcdui.game.
|